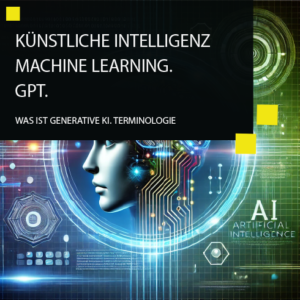
Was ist generative KI: Terminologie
Einführung Generative KI ist ein dynamisches und sich schnell entwickelndes Feld innerhalb der künstlichen Intelligenz. Es konzentriert sich auf die Entwicklung von Algorithmen, die neuartige
This white paper explores Java Reactive Programming, highlighting its advantages, disadvantages, and comparisons with traditional programming paradigms. It delves into the best use cases for reactive programming, provides example projects for getting started, and discusses essential frameworks like Quarkus, including necessary dependencies.
Java Reactive Programming represents a paradigm shift in software development, emphasizing asynchronous data streams and non-blocking operations. This approach addresses the limitations of traditional programming in handling concurrent operations, providing enhanced performance, scalability, and resilience. The increasing demand for real-time, high-throughput applications has propelled reactive programming into the spotlight, making it a critical skill for modern developers.
Reactive Streams – the new Streams‘ Standard is a key feature in understanding and implementing reactive programming in the context of Quarkus. It plays a significant role in developing highly responsive systems, leveraging the java capabilities to handle asynchronous data streams efficiently.
Traditional programming models often struggle with the complexity of handling asynchronous tasks efficiently. Blocking operations, thread management, and resource contention are usual challenges that can lead to inferior performance and scalability issues. Reactive programming offers a solution by promoting a declarative style of handling asynchronous events, reducing complexity, and improving resource utilization.
Reactive programming is based on the principles outlined in the Reactive Manifesto, which emphasizes responsiveness, resilience, elasticity, and message-driven communication. Java, with its rich ecosystem, supports reactive programming through libraries and frameworks such as Project Reactor, Akka, and Vert.x. Among these, Quarkus has gained prominence for its ability to streamline the development of reactive applications.
Modern businesses require a new type of system that can remain responsive at all times, specifically in relation to reactive programming and the development of highly responsive systems with the Quarkus Framework.
Improved Performance: Reactive programming leverages non-blocking I/O operations, which can handle more concurrent requests with fewer resources, leading to better performance and reduced latency.
Scalability: Reactive systems can scale horizontally, handling an increasing number of users and data volumes without significant changes to the codebase.
Resilience: By designing systems to handle failures gracefully through mechanisms like circuit breakers and backpressure, reactive programming ensures higher availability and reliability.
Resource Efficiency: Reactive applications make efficient use of system resources, such as CPU and memory, by avoiding unnecessary blocking and waiting, thus optimizing overall resource consumption.
Complexity: The shift to reactive programming requires a steep learning curve. Developers must understand concepts like observables, backpressure, and non-blocking I/O, which can be challenging for those accustomed to imperative programming.
Debugging and Tracing: Asynchronous code can be harder to debug and trace compared to synchronous code. Tools and techniques for effective debugging are still evolving.
Library and Ecosystem Maturity: While the ecosystem for reactive programming in Java is robust, it is not as mature as traditional programming frameworks. This can lead to compatibility issues and limited support for some libraries.
Execution Model: Traditional programming typically uses blocking I/O and thread-per-request models, leading to potential bottlenecks. In contrast, reactive programming uses non-blocking I/O and event-driven models, which can handle many more requests concurrently. This approach is particularly beneficial for creating and securing web applications based on Quarkus microservices applications.
Concurrency: Regular programming often relies on explicit thread management and synchronization mechanisms, which can be error-prone and inefficient. Reactive programming abstracts these details, offering a more declarative approach to concurrency.
Error Handling: Traditional error handling involves try-catch blocks and manual propagation of errors. Reactive programming provides more sophisticated error handling strategies, such as fallback methods, retries, and circuit breakers.
Quelle: https://mostafa-asg.github.io/post/reactive-systems-vs-reactive-programming/Core Concepts
At the heart of reactive programming is the concept of asynchronous data streams. Unlike traditional synchronous programming, where operations block and wait for data, asynchronous streams allow data to flow freely without blocking the execution thread. This non-blocking behavior ensures that system resources are used more efficiently, allowing multiple operations to be processed concurrently.
Non-blocking I/O is a key feature of reactive programming. It allows a single thread to manage multiple I/O operations simultaneously without getting blocked. This approach contrasts with the conventional thread-per-request model, where each request occupies a separate thread, leading to potential bottlenecks and inefficiencies in resource utilization.
Backpressure is a mechanism used to manage the flow of data between producers and consumers. In a reactive system, data producers can generate data faster than consumers can process it. Backpressure strategies help ensure that consumers are not overwhelmed by the data flow, maintaining system stability and performance. This is achieved through techniques like buffering, throttling, and dropping data when necessary.
Reactive programming relies on the observer pattern, where observables (data sources) emit items and subscribers (data consumers) react to these emissions. This pattern decouples the production and consumption of data, allowing for more flexible and dynamic handling of asynchronous events. Observables can represent anything from simple data values to complex event streams, providing a versatile foundation for reactive applications.
Uni represents a single value or no value at all. It is similar to CompletableFuture or Optional but specifically designed for reactive programming. Uni is useful for operations that produce a single result or may complete without producing a result (such as asynchronous operations).
Creating a Uni
A Uni can be created from a value, failure, or asynchronous computation
Different ways of creating a Uni
Consuming a Uni
A Uni can be subscribed to, to react to its completion
Consuming a Uni with Subscription
Transforming a Uni
Transformations can be applied to the result of a Uni, using operations like map, flatMap, onItem, and onFailure.
Transforming a Uni with Map
Multi represents a stream of values, which can be finite or infinite. It is similar to Publisher from the Reactive Streams specification but tailored for the needs of reactive programming in Quarkus.
Creating a Multi
A Uni can be created from a range of values, an iterable, or an asynchronous emitter
Different ways of creating a Multi
Consuming a Multi
A Multi can be subscribed to, to react to each emitted item
Consuming a Multi with Subscription
Transforming a Uni
Transformations can be applied to the stream of a Multi, using operations like map, flatMap, onItem, and onFailure.
Transforming a Multi with Transform
To demonstrate reactive programming in a practical context, we will build a simple API in Quarkus that handles both single-value and stream responses using Uni and Multi. This example will cover the service and resource layers to provide a simplified example of how to implement reactive APIs in Quarkus.
Service Layer
The service layer contains the business logic. Here, we will create a GreetingService class that provides methods returning Uni and Multi.
Simple Reactive Service Layer Example
Resource Layer
The resource layer defines the RESTful endpoints. We will create a GreetingResource class that uses the GreetingService to handle HTTP requests.
Simple Reactive Resource Layer Example
This simplified approach should illustrate how to create a simple API using Reactive components. From this point the application can be expanded to include real business logic, integrations with other systems and databases.
Real-Time Data Processing: Applications that require real-time processing of data streams, such as stock trading platforms and IoT systems.
High-Concurrency Applications: Systems with a high number of simultaneous users or connections, like social media platforms and online gaming servers.
Microservices Architectures: Distributed systems where components need to communicate asynchronously and handle high loads efficiently.
Resource-Constrained Environments: Applications running on limited resources, where efficient use of CPU and memory is crucial.
Reactive REST API: Create a RESTful API using Spring WebFlux or Quarkus to handle high loads efficiently.
Streaming Data Application: Build an application using Project Reactor or Akka Streams to process continuous streams of data in real-time.
Event-Driven Microservices: Develop a microservices-based architecture with Vert.x or Quarkus, focusing on asynchronous communication and resilience.
Quarkus is a Kubernetes-native Java framework tailored for GraalVM and OpenJDK HotSpot, making it an excellent choice for developing reactive applications. To get started with Quarkus and reactive programming, you need the following dependencies:
Standard Quarkus Reactive Dependencies
Start Small: Begin with small, manageable projects to get familiar with reactive programming concepts and gradually move to more complex applications.
Utilize the Right Tools: Make use of reactive-friendly tools and libraries such as Spring WebFlux, Project Reactor, Akka, and Vert.x.
Understand Backpressure: Learn about backpressure and how to manage it effectively to prevent overloading your system.
Leverage Community Resources: Participate in community forums, read blogs, and attend webinars to stay updated with the latest trends and best practices in reactive programming.
For developers interested in delving deeper into Java Reactive Programming and mastering its concepts and applications, here are some recommended next steps, including resources and tutorials to enhance your knowledge and skills:
Quarkus Official Documentation: The official Quarkus documentation provides comprehensive guides and references on building reactive applications.
Quarkus Reactive Guide
SmallRye Mutiny Documentation: SmallRye Mutiny is the reactive programming library used by Quarkus. Its documentation offers detailed explanations and examples.
SmallRye Mutiny Documentation
Reactive Programming with Java: A hands-on tutorial by Baeldung that covers the basics of reactive programming in Java, including Project Reactor and Spring WebFlux.
Reactive Programming with Java
Quarkus Reactive Workshops: Interactive workshops and tutorials provided by the Quarkus team to help you get started with reactive programming.
Quarkus Workshops
“Reactive Programming with RxJava” by Tomasz Nurkiewicz and Ben Christensen: This book is for Java developers who want to develop robust and reactive applications that can scale in the cloud. It provides a deep dive into reactive programming with RxJava. Reactive Programming with RxJava
“Hands-On Reactive Programming in Spring 5” by Oleh Dokuka and Igor Lozynskyi: This book is for Java developers who use Spring to develop robust and reactive applications that can scale in the cloud. It covers reactive programming with Spring 5 and Project Reactor. Hands-On Reactive Programming in Spring 5
“Reactive Systems Architecture” by Jonas Bonér: An article that explores the principles of reactive systems architecture and design. Reactive Systems Architecture
Quarkus Quickstarts: Explore a variety of example projects provided by the Quarkus team to see how different reactive concepts are implemented.
Quarkus Quickstarts
Project Reactor Examples: A collection of examples demonstrating the use of Project Reactor in various scenarios.
Project Reactor Hands-On
Java Reactive Programming offers a powerful paradigm for building responsive, resilient, and scalable applications. While it presents a learning curve and some challenges, its benefits in handling concurrency and resource efficiency are substantial. Frameworks like Quarkus simplify the development of reactive applications, providing robust tools and libraries to get started. As the demand for high-performance, real-time applications grows, mastering reactive programming becomes increasingly valuable for modern developers.
It is our hope that this article provided an helpful overview on how to approach reactive programming with Quarkus, or an alternate tool. Software development is an ever changing landscape, and keeping up-to-date with technologies and methodologies for developing sturdier, more reliable and stable applications should be the goal for all developers.
Oh and don’t forget to read our article on Problem Driven Development.
„Reactive Manifesto.“ Reactive Manifesto. https://www.reactivemanifesto.org/
„Project Reactor.“ Project Reactor. https://projectreactor.io/
„Quarkus.“ Quarkus. https://quarkus.io/
„Spring WebFlux.“ Spring WebFlux. https://spring.io/projects/spring-webflux
„Akka.“ Akka.https://akka.io/
Einführung Generative KI ist ein dynamisches und sich schnell entwickelndes Feld innerhalb der künstlichen Intelligenz. Es konzentriert sich auf die Entwicklung von Algorithmen, die neuartige
Der Kunde. Die Bank eines großen deutschen Automotive OEMs wickelt Leasing und andere Finanzierungsmodelle des OEMs ab. Sie unterliegt der Deutschen sowie der Europäischen Bankenaufsicht und
Der Kunde. Das Ministerium des Inneren des Landes Brandenburg / MIK trägt eine Vielzahl zentraler Aufgaben, die von Querschnittsfunktionen wie Organisation und Vermessungsangelegenheiten bis hin
Der Kunde. Die PD – Berater der öffentlichen Hand GmbH ist eine Partnerschaftsgesellschaft, die sich auf die Beratung öffentlicher Institutionen spezialisiert hat. Als Inhouse-Gesellschaft unterstützt
Der Kunde. CARIAD ist die Software- und Technologieeinheit des Volkswagen-Konzerns, die 2020 gegründet wurde, um die Digitalisierung und Vernetzung von Fahrzeugen voranzutreiben. Mit Fokus auf
Der Kunde. Die Volkswagen AG ist ein weltweit führender Automobilhersteller, der eine breite Palette von Fahrzeugen unter verschiedenen Marken wie Volkswagen, Audi und Porsche produziert.
Sie haben konkrete Fragen oder wollen unser Portfolio kennenlernen? Geben Sie uns Bescheid und wir rufen Sie zu Ihrem Wunschtermin zurück!
Sie können uns auch gerne direkt anrufen.